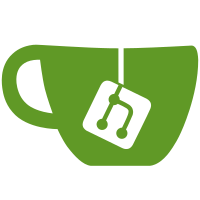
can now handle that; this allows us to register both the modulo-8 and the modulo-128 versions of various X.25 bitfields with "x.25.XXX" names, which lets us get rid of the "ex.25" protocol stuff completely and use "x.25" for both modulo-8 and modulo-128 X.25. Do so. (Also, fix up some cases where we appeared to be using the modulo-8 fields when dissecting modulo-128 X.25.) This, in turn, allows us to register the X.25 dissector, as there's now only one protocol with which it's associated, and make it static and have it called only through a handle, and to, when registering it with the "llc.dsap" dissector table, associate it with "proto_x25". That, in turn, allows us to get rid of the "CHECK_DISPLAY_AS_DATA()" calls, and the code to set "pinfo->current_proto", in the X.25 dissector. The code for the display filter expression dialog would, if there are two fields with the same name registered under a protocol, list both of them; have it list only one of them - the fields should have the same type, the same radix, and the same value_string/true_false_string table if any (if they don't, they're really not the same field...). svn path=/trunk/; revision=3023
104 lines
2.9 KiB
C
104 lines
2.9 KiB
C
/* packet-xot.c
|
|
* Routines for X25 over TCP dissection (RFC 1613)
|
|
*
|
|
* Copyright 2000, Paul Ionescu <paul@acorp.ro>
|
|
*
|
|
* $Id: packet-xot.c,v 1.5 2001/02/12 09:06:17 guy Exp $
|
|
*
|
|
* Ethereal - Network traffic analyzer
|
|
* By Gerald Combs <gerald@zing.org>
|
|
* Copyright 1998 Gerald Combs
|
|
*
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
# include "config.h"
|
|
#endif
|
|
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <ctype.h>
|
|
|
|
#include <string.h>
|
|
#include <glib.h>
|
|
#include "packet.h"
|
|
|
|
#define TCP_PORT_XOT 1998
|
|
|
|
static gint proto_xot = -1;
|
|
static gint ett_xot = -1;
|
|
|
|
static dissector_handle_t x25_handle;
|
|
|
|
static void dissect_xot(tvbuff_t *tvb, packet_info *pinfo, proto_tree *tree)
|
|
{
|
|
proto_item *ti;
|
|
proto_tree *xot_tree;
|
|
guint16 version,len;
|
|
tvbuff_t *next_tvb;
|
|
|
|
if (check_col(pinfo->fd, COL_PROTOCOL))
|
|
col_set_str(pinfo->fd, COL_PROTOCOL, "XOT");
|
|
if (check_col(pinfo->fd, COL_INFO))
|
|
col_clear(pinfo->fd, COL_INFO);
|
|
|
|
version = tvb_get_ntohs(tvb,0);
|
|
len = tvb_get_ntohs(tvb,2);
|
|
|
|
if (check_col(pinfo->fd, COL_INFO))
|
|
col_add_fstr(pinfo->fd, COL_INFO, "XOT Version = %u, size = %u",version,len );
|
|
|
|
if (tree) {
|
|
|
|
ti = proto_tree_add_protocol_format(tree, proto_xot, tvb, 0, 4, "X.25 over TCP");
|
|
xot_tree = proto_item_add_subtree(ti, ett_xot);
|
|
|
|
ti = proto_tree_add_text(xot_tree, tvb, 0,2,"XOT Version : %u %s",version,(version==0?"":" - Unknown version")) ;
|
|
ti = proto_tree_add_text(xot_tree, tvb, 2,2,"XOT length : %u",len) ;
|
|
}
|
|
next_tvb = tvb_new_subset(tvb,4, -1 , -1);
|
|
call_dissector(x25_handle,next_tvb,pinfo,tree);
|
|
}
|
|
|
|
/* Register the protocol with Ethereal */
|
|
void proto_register_xot(void)
|
|
{
|
|
|
|
|
|
/* Setup protocol subtree array */
|
|
static gint *ett[] = {
|
|
&ett_xot,
|
|
};
|
|
|
|
/* Register the protocol name and description */
|
|
proto_xot = proto_register_protocol("X.25 over TCP", "XOT", "xot");
|
|
|
|
/* Required function calls to register the header fields and subtrees used */
|
|
proto_register_subtree_array(ett, array_length(ett));
|
|
};
|
|
|
|
void
|
|
proto_reg_handoff_xot(void)
|
|
{
|
|
/*
|
|
* Get a handle for the X.25 dissector.
|
|
*/
|
|
x25_handle = find_dissector("x.25");
|
|
|
|
dissector_add("tcp.port", TCP_PORT_XOT, dissect_xot, proto_xot);
|
|
}
|