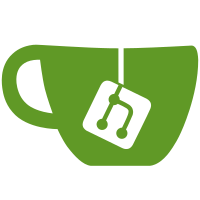
When copying hexdumps, the newline might be missing which would result in a capture file missing one byte in its packet. Adjust the grammar to recognize the two trailing hexadecimal characters as a "byte". This is safe because Flex picks the rule that matches the longest input string. So given "01 ", it will always match all three characters. If something like "01x" is given, then the "text" rule will be matched (as before). Only if no more characters are available (such as at the end of a file), then the rule will match two hexdigits. Remove the unnecessary hexdigit rule while at it. Change-Id: I21dc37d684d1c410ce720cb27706a6e54f87f94d Reviewed-on: https://code.wireshark.org/review/30190 Petri-Dish: Peter Wu <peter@lekensteyn.nl> Tested-by: Petri Dish Buildbot Reviewed-by: Anders Broman <a.broman58@gmail.com>
113 lines
2.8 KiB
Text
113 lines
2.8 KiB
Text
/* -*-mode: flex-*- */
|
|
|
|
%top {
|
|
/* Include this before everything else, for various large-file definitions */
|
|
#include "config.h"
|
|
}
|
|
|
|
/*
|
|
* We don't use input, so don't generate code for it.
|
|
*/
|
|
%option noinput
|
|
|
|
/*
|
|
* We don't use unput, so don't generate code for it.
|
|
*/
|
|
%option nounput
|
|
|
|
/*
|
|
* We don't read interactively from the terminal.
|
|
*/
|
|
%option never-interactive
|
|
|
|
/*
|
|
* We want to stop processing when we get to the end of the input.
|
|
*/
|
|
%option noyywrap
|
|
|
|
/*
|
|
* Prefix scanner routines with "text2pcap_" rather than "yy" to avoid a
|
|
* "redefined macro" warning with flex 2.6.3.
|
|
*/
|
|
%option prefix="text2pcap_"
|
|
|
|
%{
|
|
|
|
/********************************************************************************
|
|
*
|
|
* text2pcap-scanner.l
|
|
*
|
|
* Utility to convert an ASCII hexdump into a libpcap-format capture file
|
|
*
|
|
* (c) Copyright 2001 Ashok Narayanan <ashokn@cisco.com>
|
|
*
|
|
* Wireshark - Network traffic analyzer
|
|
* By Gerald Combs <gerald@wireshark.org>
|
|
* Copyright 1998 Gerald Combs
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0-or-later
|
|
*
|
|
*******************************************************************************/
|
|
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
|
|
#include "text2pcap.h"
|
|
|
|
/*
|
|
* Disable diagnostics in the code generated by Flex.
|
|
*/
|
|
DIAG_OFF_FLEX
|
|
|
|
/*
|
|
* Flex (v 2.5.35) uses this symbol to "exclude" unistd.h
|
|
*/
|
|
#ifdef _WIN32
|
|
#define YY_NO_UNISTD_H
|
|
#endif
|
|
|
|
%}
|
|
|
|
directive ^#TEXT2PCAP.*\r?\n
|
|
comment ^[\t ]*#.*\r?\n
|
|
byte [0-9A-Fa-f][0-9A-Fa-f][ \t]?
|
|
byte_eol [0-9A-Fa-f][0-9A-Fa-f]\r?\n
|
|
offset [0-9A-Fa-f]+[: \t]
|
|
offset_eol [0-9A-Fa-f]+\r?\n
|
|
text [^ \n\t]+
|
|
mailfwd >
|
|
eol \r?\n\r?
|
|
|
|
%%
|
|
|
|
{byte} { if (parse_token(T_BYTE, yytext) != EXIT_SUCCESS) return EXIT_FAILURE; }
|
|
{byte_eol} { if (parse_token(T_BYTE, yytext) != EXIT_SUCCESS) return EXIT_FAILURE;
|
|
if (parse_token(T_EOL, NULL) != EXIT_SUCCESS) return EXIT_FAILURE; }
|
|
{offset} { if (parse_token(T_OFFSET, yytext) != EXIT_SUCCESS) return EXIT_FAILURE; }
|
|
{offset_eol} { if (parse_token(T_OFFSET, yytext) != EXIT_SUCCESS) return EXIT_FAILURE;
|
|
if (parse_token(T_EOL, NULL) != EXIT_SUCCESS) return EXIT_FAILURE; }
|
|
{mailfwd}{offset} { if (parse_token(T_OFFSET, yytext+1) != EXIT_SUCCESS) return EXIT_FAILURE; }
|
|
{eol} { if (parse_token(T_EOL, NULL) != EXIT_SUCCESS) return EXIT_FAILURE; }
|
|
[ \t] ; /* ignore whitespace */
|
|
{directive} { if (parse_token(T_DIRECTIVE, yytext) != EXIT_SUCCESS) return EXIT_FAILURE;
|
|
if (parse_token(T_EOL, NULL) != EXIT_SUCCESS) return EXIT_FAILURE; }
|
|
{comment} { if (parse_token(T_EOL, NULL) != EXIT_SUCCESS) return EXIT_FAILURE; }
|
|
{text} { if (parse_token(T_TEXT, yytext) != EXIT_SUCCESS) return EXIT_FAILURE; }
|
|
|
|
%%
|
|
|
|
/*
|
|
* Turn diagnostics back on, so we check the code that we've written.
|
|
*/
|
|
DIAG_ON_FLEX
|
|
|
|
int
|
|
text2pcap_scan(void)
|
|
{
|
|
int ret;
|
|
|
|
ret = text2pcap_lex();
|
|
text2pcap_lex_destroy();
|
|
return ret;
|
|
}
|