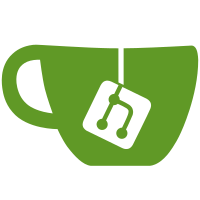
The Linux SocketCAN header now uses the formerly-reserved byte in the SocketCAN header after the "payload length" field as an "FD flags" field, with a flag bit reserved to indicate whether the frame is a classic CAN frame or a CAN FD frame, with two other bits giving frame information for FD frames. For LINKTYPE_CAN_SOCKETCAN, use that flag bit to determine whether the frame is classic CAN or CAN FD. As some older LINKTYPE_CAN_SOCKETCAN captures have SocketCAN headers in which the fields after the "payload length" field were uninitialized, so trust that thge "FD flags" was filled in, rather than possibly randomly uninitialized, only if the only bits set in that field are the bits defined to be in that field and the two reserved bytes after it are zero. This will be needed when the current main-branch libpcap is released, as it uses LINKTYPE_CAN_SOCKETCAN rather than LINKTYPE_LINUX_SLL for ARPHRD_CAN devices; we add it now to future-proof the Wireshark releases to which this is being committed. It also handles what existing CAN FD captures using LINKTYPE_CAN_SOCKETCAN exist. For LINKTYPE_LINUX_SLL frames, we have the protocol field to distinguish between classic CAN and CAN FD, so we use that to determine the frame type, rather than looking at the CANFD_FDF flag. dissect_socketcan_common() now handles both classic CAN and CAN FD frames.
79 lines
2.7 KiB
C
79 lines
2.7 KiB
C
/* packet-socketcan.h
|
|
*
|
|
* Wireshark - Network traffic analyzer
|
|
* By Gerald Combs <gerald@wireshark.org>
|
|
* Copyright 1998 Gerald Combs
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0-or-later
|
|
*/
|
|
|
|
#ifndef __PACKET_SOCKETCAN_H__
|
|
#define __PACKET_SOCKETCAN_H__
|
|
|
|
#include <epan/tvbuff.h>
|
|
#include <epan/packet_info.h>
|
|
#include <epan/proto.h>
|
|
|
|
/* Flags for CAN FD frames. */
|
|
#define CANFD_BRS 0x01 /* Bit Rate Switch (second bitrate for payload data) */
|
|
#define CANFD_ESI 0x02 /* Error State Indicator of the transmitting node */
|
|
#define CANFD_FDF 0x04 /* FD flag - if set, this is an FD frame */
|
|
|
|
/* Structure that gets passed between dissectors. */
|
|
struct can_info
|
|
{
|
|
guint32 id;
|
|
guint32 len;
|
|
gboolean fd;
|
|
guint16 bus_id;
|
|
};
|
|
|
|
typedef struct can_info can_info_t;
|
|
|
|
/* controller area network (CAN) kernel definitions
|
|
* These masks are usually defined within <linux/can.h> but are not
|
|
* available on non-Linux platforms; that's the reason for the
|
|
* redefinitions below
|
|
*
|
|
* special address description flags for the CAN_ID */
|
|
#define CAN_EFF_FLAG 0x80000000 /* EFF/SFF is set in the MSB */
|
|
#define CAN_RTR_FLAG 0x40000000 /* remote transmission request */
|
|
#define CAN_ERR_FLAG 0x20000000 /* error frame */
|
|
|
|
#define CAN_FLAG_MASK (CAN_EFF_FLAG | CAN_RTR_FLAG | CAN_ERR_FLAG)
|
|
|
|
#define CAN_EFF_MASK 0x1FFFFFFF /* extended frame format (EFF) has a 29 bit identifier */
|
|
#define CAN_SFF_MASK 0x000007FF /* standard frame format (SFF) has a 11 bit identifier */
|
|
|
|
#define CAN_ERR_DLC 8 /* dlc for error message frames */
|
|
|
|
/* error class (mask) in can_id */
|
|
#define CAN_ERR_TX_TIMEOUT 0x00000001U /* TX timeout (by netdevice driver) */
|
|
#define CAN_ERR_LOSTARB 0x00000002U /* lost arbitration / data[0] */
|
|
#define CAN_ERR_CTRL 0x00000004U /* controller problems / data[1] */
|
|
#define CAN_ERR_PROT 0x00000008U /* protocol violations / data[2..3] */
|
|
#define CAN_ERR_TRX 0x00000010U /* transceiver status / data[4] */
|
|
#define CAN_ERR_ACK 0x00000020U /* received no ACK on transmission */
|
|
#define CAN_ERR_BUSOFF 0x00000040U /* bus off */
|
|
#define CAN_ERR_BUSERROR 0x00000080U /* bus error (may flood!) */
|
|
#define CAN_ERR_RESTARTED 0x00000100U /* controller restarted */
|
|
#define CAN_ERR_RESERVED 0x1FFFFE00U /* reserved bits */
|
|
|
|
|
|
gboolean socketcan_call_subdissectors(tvbuff_t *tvb, packet_info *pinfo, proto_tree *tree, struct can_info *can_info, const gboolean use_heuristics_first);
|
|
|
|
#endif /* __PACKET_SOCKETCAN_H__ */
|
|
|
|
/*
|
|
* Editor modelines - https://www.wireshark.org/tools/modelines.html
|
|
*
|
|
* Local variables:
|
|
* c-basic-offset: 8
|
|
* tab-width: 8
|
|
* indent-tabs-mode: t
|
|
* End:
|
|
*
|
|
* vi: set shiftwidth=8 tabstop=8 noexpandtab:
|
|
* :indentSize=8:tabSize=8:noTabs=false:
|
|
*/
|