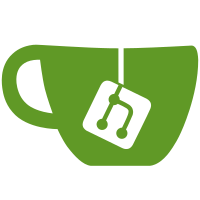
Add ColorUtils::themeLinkBrush, which returns a readable link color in dark mode. Use it in place of existing ...palette().link() calls. Add ColorUtils::themeLinkStyle, which produces an HTML <style/> block that lightens the link foreground color in dark mode. Use this to style links in the about box and in elided labels. Catch ApplicationPaletteChange events where needed. Add dark theme / dark mode notes to the WSDG. Ping-Bug: 15511 Change-Id: I92925bd997f97b155491f55a8c818f03549bc7f4 Reviewed-on: https://code.wireshark.org/review/33704 Petri-Dish: Gerald Combs <gerald@wireshark.org> Tested-by: Petri Dish Buildbot Reviewed-by: Gerald Combs <gerald@wireshark.org>
66 lines
1.6 KiB
C++
66 lines
1.6 KiB
C++
/* url_link_delegate.cpp
|
|
* Delegates for displaying links as links, including elide model
|
|
*
|
|
* Wireshark - Network traffic analyzer
|
|
* By Gerald Combs <gerald@wireshark.org>
|
|
* Copyright 1998 Gerald Combs
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0-or-later
|
|
*/
|
|
|
|
#include <ui/qt/models/url_link_delegate.h>
|
|
|
|
#include <QPainter>
|
|
#include <QRegExp>
|
|
|
|
#include <ui/qt/utils/color_utils.h>
|
|
|
|
UrlLinkDelegate::UrlLinkDelegate(QObject *parent)
|
|
: QStyledItemDelegate(parent),
|
|
re_col_(-1),
|
|
url_re_(new QRegExp())
|
|
{}
|
|
|
|
UrlLinkDelegate::~UrlLinkDelegate()
|
|
{
|
|
delete url_re_;
|
|
}
|
|
|
|
void UrlLinkDelegate::setColCheck(int column, QString &pattern)
|
|
{
|
|
re_col_ = column;
|
|
url_re_->setPattern(pattern);
|
|
}
|
|
|
|
void UrlLinkDelegate::paint(QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const {
|
|
if (re_col_ >= 0 && url_re_) {
|
|
QModelIndex re_idx = index.model()->index(index.row(), re_col_);
|
|
QString col_text = index.model()->data(re_idx).toString();
|
|
if (url_re_->indexIn(col_text) < 0) {
|
|
QStyledItemDelegate::paint(painter, option, index);
|
|
return;
|
|
}
|
|
}
|
|
|
|
QStyleOptionViewItem opt = option;
|
|
initStyleOption(&opt, index);
|
|
|
|
opt.font.setUnderline(true);
|
|
opt.palette.setColor(QPalette::Text, ColorUtils::themeLinkBrush().color());
|
|
|
|
QStyledItemDelegate::paint(painter, opt, index);
|
|
}
|
|
|
|
/*
|
|
* Editor modelines
|
|
*
|
|
* Local Variables:
|
|
* c-basic-offset: 4
|
|
* tab-width: 8
|
|
* indent-tabs-mode: nil
|
|
* End:
|
|
*
|
|
* ex: set shiftwidth=4 tabstop=8 expandtab:
|
|
* :indentSize=4:tabSize=8:noTabs=true:
|
|
*/
|