forked from osmocom/wireshark
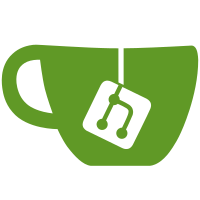
statements. Move the setting of the Protocol column in various dissectors before anything is fetched from the packet, and also clear the Info column at that point in those and some other dissectors, so that if an exception is thrown, the columns don't reflect the previous protocol. "Tvbuffify" the Mobile IP dissector (it took old-style arguments, and then converted them into tvbuff arguments, so there wasn't much to do, other than to fix references to "fd" to refer to "pinfo->fd"). In the SCTP dissector, refer to the port type and source and destination ports through "pinfo" rather than through the global "pi", as it's a tvbuffified dissector. In the SMTP and Time Protocol dissectors, use "pinfo->match_port" rather than "TCP_PORT_SMTP" when checking whether the packet is a request or reply, just in case somebody makes a non-standard port be dissected as SMTP or Time. (Also, remove a bogus comment from the Time dissector; it was probably cut-and-pasted from the TFTP dissector.) svn path=/trunk/; revision=2938
112 lines
3 KiB
C
112 lines
3 KiB
C
/* packet-lapbether.c
|
|
* Routines for lapbether frame disassembly
|
|
* Richard Sharpe <rsharpe@ns.aus.com> based on the lapb module by
|
|
* Olivier Abad <oabad@cybercable.fr>
|
|
*
|
|
* $Id: packet-lapbether.c,v 1.5 2001/01/25 06:14:14 guy Exp $
|
|
*
|
|
* Ethereal - Network traffic analyzer
|
|
* By Gerald Combs <gerald@zing.org>
|
|
* Copyright 1998
|
|
*
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
# include "config.h"
|
|
#endif
|
|
|
|
#ifdef HAVE_SYS_TYPES_H
|
|
# include <sys/types.h>
|
|
#endif
|
|
|
|
#include <stdio.h>
|
|
#include <glib.h>
|
|
#include <string.h>
|
|
#include "packet.h"
|
|
#include "etypes.h"
|
|
|
|
static int proto_lapbether = -1;
|
|
|
|
static int hf_lapbether_length = -1;
|
|
|
|
static gint ett_lapbether = -1;
|
|
|
|
static dissector_handle_t lapb_handle;
|
|
|
|
static void
|
|
dissect_lapbether(tvbuff_t *tvb, packet_info *pinfo, proto_tree *tree)
|
|
{
|
|
proto_tree *lapbether_tree, *ti;
|
|
int len;
|
|
tvbuff_t *next_tvb;
|
|
|
|
if (check_col(pinfo->fd, COL_PROTOCOL))
|
|
col_set_str(pinfo->fd, COL_PROTOCOL, "LAPBETHER");
|
|
if (check_col(pinfo->fd, COL_INFO))
|
|
col_clear(pinfo->fd, COL_INFO);
|
|
|
|
len = tvb_get_guint8(tvb, 0) + tvb_get_guint8(tvb, 1) * 256;
|
|
|
|
if (tree) {
|
|
|
|
ti = proto_tree_add_protocol_format(tree, proto_lapbether, tvb, 0, 2,
|
|
"LAPBETHER");
|
|
|
|
lapbether_tree = proto_item_add_subtree(ti, ett_lapbether);
|
|
proto_tree_add_uint_format(lapbether_tree, hf_lapbether_length, tvb, 0, 2,
|
|
len, "Length: %u", len);
|
|
|
|
}
|
|
|
|
next_tvb = tvb_new_subset(tvb, 2, len, len);
|
|
call_dissector(lapb_handle, next_tvb, pinfo, tree);
|
|
|
|
}
|
|
|
|
void
|
|
proto_register_lapbether(void)
|
|
{
|
|
static hf_register_info hf[] = {
|
|
{ &hf_lapbether_length,
|
|
{ "Length Field", "lapbether.length", FT_UINT16, BASE_DEC, NULL, 0x0,
|
|
"LAPBEther Length Field"}},
|
|
|
|
};
|
|
static gint *ett[] = {
|
|
&ett_lapbether,
|
|
};
|
|
|
|
proto_lapbether = proto_register_protocol ("Link Access Procedure Balanced Ethernet (LAPBETHER)",
|
|
"LAPBETHER", "lapbether");
|
|
proto_register_field_array (proto_lapbether, hf, array_length(hf));
|
|
proto_register_subtree_array(ett, array_length(ett));
|
|
}
|
|
|
|
/* The registration hand-off routine */
|
|
void
|
|
proto_reg_handoff_lapbether(void)
|
|
{
|
|
|
|
/*
|
|
* Get a handle for the LAPB dissector.
|
|
*/
|
|
lapb_handle = find_dissector("lapb");
|
|
|
|
dissector_add("ethertype", ETHERTYPE_DEC, dissect_lapbether, proto_lapbether);
|
|
|
|
}
|