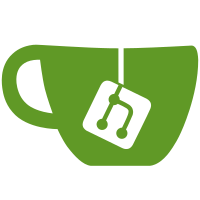
Every current transport class calls transport_container_release but ignores the return value. This is catastrophic if it returns an error because the containers are part of a global list and the next action of almost every transport class is to free the memory used by the container. Fix this by making transport_container_release a void, but making it BUG if attribute_container_release returns an error ... this catches the root cause of a system panic much earlier. If we don't do this, we get an eventual BUG when the attribute container list notices the corruption caused by the freed memory it's still referencing. Also made attribute_container_release __must_check as a reminder. Cc: Greg KH <greg@kroah.com> Signed-off-by: James Bottomley <James.Bottomley@HansenPartnership.com>
102 lines
2.5 KiB
C
102 lines
2.5 KiB
C
/*
|
|
* transport_class.h - a generic container for all transport classes
|
|
*
|
|
* Copyright (c) 2005 - James Bottomley <James.Bottomley@steeleye.com>
|
|
*
|
|
* This file is licensed under GPLv2
|
|
*/
|
|
|
|
#ifndef _TRANSPORT_CLASS_H_
|
|
#define _TRANSPORT_CLASS_H_
|
|
|
|
#include <linux/device.h>
|
|
#include <linux/attribute_container.h>
|
|
|
|
struct transport_container;
|
|
|
|
struct transport_class {
|
|
struct class class;
|
|
int (*setup)(struct transport_container *, struct device *,
|
|
struct class_device *);
|
|
int (*configure)(struct transport_container *, struct device *,
|
|
struct class_device *);
|
|
int (*remove)(struct transport_container *, struct device *,
|
|
struct class_device *);
|
|
};
|
|
|
|
#define DECLARE_TRANSPORT_CLASS(cls, nm, su, rm, cfg) \
|
|
struct transport_class cls = { \
|
|
.class = { \
|
|
.name = nm, \
|
|
}, \
|
|
.setup = su, \
|
|
.remove = rm, \
|
|
.configure = cfg, \
|
|
}
|
|
|
|
|
|
struct anon_transport_class {
|
|
struct transport_class tclass;
|
|
struct attribute_container container;
|
|
};
|
|
|
|
#define DECLARE_ANON_TRANSPORT_CLASS(cls, mtch, cfg) \
|
|
struct anon_transport_class cls = { \
|
|
.tclass = { \
|
|
.configure = cfg, \
|
|
}, \
|
|
. container = { \
|
|
.match = mtch, \
|
|
}, \
|
|
}
|
|
|
|
#define class_to_transport_class(x) \
|
|
container_of(x, struct transport_class, class)
|
|
|
|
struct transport_container {
|
|
struct attribute_container ac;
|
|
struct attribute_group *statistics;
|
|
};
|
|
|
|
#define attribute_container_to_transport_container(x) \
|
|
container_of(x, struct transport_container, ac)
|
|
|
|
void transport_remove_device(struct device *);
|
|
void transport_add_device(struct device *);
|
|
void transport_setup_device(struct device *);
|
|
void transport_configure_device(struct device *);
|
|
void transport_destroy_device(struct device *);
|
|
|
|
static inline void
|
|
transport_register_device(struct device *dev)
|
|
{
|
|
transport_setup_device(dev);
|
|
transport_add_device(dev);
|
|
}
|
|
|
|
static inline void
|
|
transport_unregister_device(struct device *dev)
|
|
{
|
|
transport_remove_device(dev);
|
|
transport_destroy_device(dev);
|
|
}
|
|
|
|
static inline int transport_container_register(struct transport_container *tc)
|
|
{
|
|
return attribute_container_register(&tc->ac);
|
|
}
|
|
|
|
static inline void transport_container_unregister(struct transport_container *tc)
|
|
{
|
|
if (unlikely(attribute_container_unregister(&tc->ac)))
|
|
BUG();
|
|
}
|
|
|
|
int transport_class_register(struct transport_class *);
|
|
int anon_transport_class_register(struct anon_transport_class *);
|
|
void transport_class_unregister(struct transport_class *);
|
|
void anon_transport_class_unregister(struct anon_transport_class *);
|
|
|
|
|
|
#endif
|