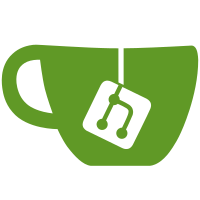
Create a kernel/rcutree_plugin.h file that contains definitions for preemptable RCU (or, under the #else branch of the #ifdef, empty definitions for the classic non-preemptable semantics). These definitions fit into plugins defined in kernel/rcutree.c for this purpose. This variant of preemptable RCU uses a new algorithm whose read-side expense is roughly that of classic hierarchical RCU under CONFIG_PREEMPT. This new algorithm's update-side expense is similar to that of classic hierarchical RCU, and, in absence of read-side preemption or blocking, is exactly that of classic hierarchical RCU. Perhaps more important, this new algorithm has a much simpler implementation, saving well over 1,000 lines of code compared to mainline's implementation of preemptable RCU, which will hopefully be retired in favor of this new algorithm. The simplifications are obtained by maintaining per-task nesting state for running tasks, and using a simple lock-protected algorithm to handle accounting when tasks block within RCU read-side critical sections, making use of lessons learned while creating numerous user-level RCU implementations over the past 18 months. Signed-off-by: Paul E. McKenney <paulmck@linux.vnet.ibm.com> Cc: laijs@cn.fujitsu.com Cc: dipankar@in.ibm.com Cc: akpm@linux-foundation.org Cc: mathieu.desnoyers@polymtl.ca Cc: josht@linux.vnet.ibm.com Cc: dvhltc@us.ibm.com Cc: niv@us.ibm.com Cc: peterz@infradead.org Cc: rostedt@goodmis.org LKML-Reference: <12509746134003-git-send-email-> Signed-off-by: Ingo Molnar <mingo@elte.hu>
117 lines
2.8 KiB
C
117 lines
2.8 KiB
C
/*
|
|
* Read-Copy Update mechanism for mutual exclusion (tree-based version)
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
*
|
|
* Copyright IBM Corporation, 2008
|
|
*
|
|
* Author: Dipankar Sarma <dipankar@in.ibm.com>
|
|
* Paul E. McKenney <paulmck@linux.vnet.ibm.com> Hierarchical algorithm
|
|
*
|
|
* Based on the original work by Paul McKenney <paulmck@us.ibm.com>
|
|
* and inputs from Rusty Russell, Andrea Arcangeli and Andi Kleen.
|
|
*
|
|
* For detailed explanation of Read-Copy Update mechanism see -
|
|
* Documentation/RCU
|
|
*/
|
|
|
|
#ifndef __LINUX_RCUTREE_H
|
|
#define __LINUX_RCUTREE_H
|
|
|
|
extern void rcu_sched_qs(int cpu);
|
|
extern void rcu_bh_qs(int cpu);
|
|
|
|
extern int rcu_needs_cpu(int cpu);
|
|
|
|
#ifdef CONFIG_TREE_PREEMPT_RCU
|
|
|
|
extern void __rcu_read_lock(void);
|
|
extern void __rcu_read_unlock(void);
|
|
extern void exit_rcu(void);
|
|
|
|
#else /* #ifdef CONFIG_TREE_PREEMPT_RCU */
|
|
|
|
static inline void __rcu_read_lock(void)
|
|
{
|
|
preempt_disable();
|
|
}
|
|
|
|
static inline void __rcu_read_unlock(void)
|
|
{
|
|
preempt_enable();
|
|
}
|
|
|
|
static inline void exit_rcu(void)
|
|
{
|
|
}
|
|
|
|
#endif /* #else #ifdef CONFIG_TREE_PREEMPT_RCU */
|
|
|
|
static inline void __rcu_read_lock_bh(void)
|
|
{
|
|
local_bh_disable();
|
|
}
|
|
static inline void __rcu_read_unlock_bh(void)
|
|
{
|
|
local_bh_enable();
|
|
}
|
|
|
|
#define __synchronize_sched() synchronize_rcu()
|
|
|
|
extern void call_rcu_sched(struct rcu_head *head,
|
|
void (*func)(struct rcu_head *rcu));
|
|
|
|
static inline void synchronize_rcu_expedited(void)
|
|
{
|
|
synchronize_sched_expedited();
|
|
}
|
|
|
|
static inline void synchronize_rcu_bh_expedited(void)
|
|
{
|
|
synchronize_sched_expedited();
|
|
}
|
|
|
|
extern void __rcu_init(void);
|
|
extern void rcu_check_callbacks(int cpu, int user);
|
|
extern void rcu_restart_cpu(int cpu);
|
|
|
|
extern long rcu_batches_completed(void);
|
|
extern long rcu_batches_completed_bh(void);
|
|
extern long rcu_batches_completed_sched(void);
|
|
|
|
static inline void rcu_init_sched(void)
|
|
{
|
|
}
|
|
|
|
#ifdef CONFIG_NO_HZ
|
|
void rcu_enter_nohz(void);
|
|
void rcu_exit_nohz(void);
|
|
#else /* CONFIG_NO_HZ */
|
|
static inline void rcu_enter_nohz(void)
|
|
{
|
|
}
|
|
static inline void rcu_exit_nohz(void)
|
|
{
|
|
}
|
|
#endif /* CONFIG_NO_HZ */
|
|
|
|
/* A context switch is a grace period for rcutree. */
|
|
static inline int rcu_blocking_is_gp(void)
|
|
{
|
|
return num_online_cpus() == 1;
|
|
}
|
|
|
|
#endif /* __LINUX_RCUTREE_H */
|