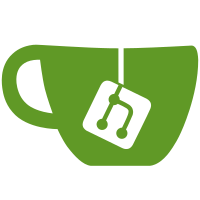
The kernel's implementation of notifier chains is unsafe. There is no protection against entries being added to or removed from a chain while the chain is in use. The issues were discussed in this thread: http://marc.theaimsgroup.com/?l=linux-kernel&m=113018709002036&w=2 We noticed that notifier chains in the kernel fall into two basic usage classes: "Blocking" chains are always called from a process context and the callout routines are allowed to sleep; "Atomic" chains can be called from an atomic context and the callout routines are not allowed to sleep. We decided to codify this distinction and make it part of the API. Therefore this set of patches introduces three new, parallel APIs: one for blocking notifiers, one for atomic notifiers, and one for "raw" notifiers (which is really just the old API under a new name). New kinds of data structures are used for the heads of the chains, and new routines are defined for registration, unregistration, and calling a chain. The three APIs are explained in include/linux/notifier.h and their implementation is in kernel/sys.c. With atomic and blocking chains, the implementation guarantees that the chain links will not be corrupted and that chain callers will not get messed up by entries being added or removed. For raw chains the implementation provides no guarantees at all; users of this API must provide their own protections. (The idea was that situations may come up where the assumptions of the atomic and blocking APIs are not appropriate, so it should be possible for users to handle these things in their own way.) There are some limitations, which should not be too hard to live with. For atomic/blocking chains, registration and unregistration must always be done in a process context since the chain is protected by a mutex/rwsem. Also, a callout routine for a non-raw chain must not try to register or unregister entries on its own chain. (This did happen in a couple of places and the code had to be changed to avoid it.) Since atomic chains may be called from within an NMI handler, they cannot use spinlocks for synchronization. Instead we use RCU. The overhead falls almost entirely in the unregister routine, which is okay since unregistration is much less frequent that calling a chain. Here is the list of chains that we adjusted and their classifications. None of them use the raw API, so for the moment it is only a placeholder. ATOMIC CHAINS ------------- arch/i386/kernel/traps.c: i386die_chain arch/ia64/kernel/traps.c: ia64die_chain arch/powerpc/kernel/traps.c: powerpc_die_chain arch/sparc64/kernel/traps.c: sparc64die_chain arch/x86_64/kernel/traps.c: die_chain drivers/char/ipmi/ipmi_si_intf.c: xaction_notifier_list kernel/panic.c: panic_notifier_list kernel/profile.c: task_free_notifier net/bluetooth/hci_core.c: hci_notifier net/ipv4/netfilter/ip_conntrack_core.c: ip_conntrack_chain net/ipv4/netfilter/ip_conntrack_core.c: ip_conntrack_expect_chain net/ipv6/addrconf.c: inet6addr_chain net/netfilter/nf_conntrack_core.c: nf_conntrack_chain net/netfilter/nf_conntrack_core.c: nf_conntrack_expect_chain net/netlink/af_netlink.c: netlink_chain BLOCKING CHAINS --------------- arch/powerpc/platforms/pseries/reconfig.c: pSeries_reconfig_chain arch/s390/kernel/process.c: idle_chain arch/x86_64/kernel/process.c idle_notifier drivers/base/memory.c: memory_chain drivers/cpufreq/cpufreq.c cpufreq_policy_notifier_list drivers/cpufreq/cpufreq.c cpufreq_transition_notifier_list drivers/macintosh/adb.c: adb_client_list drivers/macintosh/via-pmu.c sleep_notifier_list drivers/macintosh/via-pmu68k.c sleep_notifier_list drivers/macintosh/windfarm_core.c wf_client_list drivers/usb/core/notify.c usb_notifier_list drivers/video/fbmem.c fb_notifier_list kernel/cpu.c cpu_chain kernel/module.c module_notify_list kernel/profile.c munmap_notifier kernel/profile.c task_exit_notifier kernel/sys.c reboot_notifier_list net/core/dev.c netdev_chain net/decnet/dn_dev.c: dnaddr_chain net/ipv4/devinet.c: inetaddr_chain It's possible that some of these classifications are wrong. If they are, please let us know or submit a patch to fix them. Note that any chain that gets called very frequently should be atomic, because the rwsem read-locking used for blocking chains is very likely to incur cache misses on SMP systems. (However, if the chain's callout routines may sleep then the chain cannot be atomic.) The patch set was written by Alan Stern and Chandra Seetharaman, incorporating material written by Keith Owens and suggestions from Paul McKenney and Andrew Morton. [jes@sgi.com: restructure the notifier chain initialization macros] Signed-off-by: Alan Stern <stern@rowland.harvard.edu> Signed-off-by: Chandra Seetharaman <sekharan@us.ibm.com> Signed-off-by: Jes Sorensen <jes@sgi.com> Signed-off-by: Andrew Morton <akpm@osdl.org> Signed-off-by: Linus Torvalds <torvalds@osdl.org>
391 lines
11 KiB
C
391 lines
11 KiB
C
#ifndef _IP_CONNTRACK_H
|
|
#define _IP_CONNTRACK_H
|
|
|
|
#include <linux/netfilter/nf_conntrack_common.h>
|
|
|
|
#ifdef __KERNEL__
|
|
#include <linux/config.h>
|
|
#include <linux/netfilter_ipv4/ip_conntrack_tuple.h>
|
|
#include <linux/bitops.h>
|
|
#include <linux/compiler.h>
|
|
#include <asm/atomic.h>
|
|
|
|
#include <linux/netfilter_ipv4/ip_conntrack_tcp.h>
|
|
#include <linux/netfilter_ipv4/ip_conntrack_icmp.h>
|
|
#include <linux/netfilter_ipv4/ip_conntrack_proto_gre.h>
|
|
#include <linux/netfilter_ipv4/ip_conntrack_sctp.h>
|
|
|
|
/* per conntrack: protocol private data */
|
|
union ip_conntrack_proto {
|
|
/* insert conntrack proto private data here */
|
|
struct ip_ct_gre gre;
|
|
struct ip_ct_sctp sctp;
|
|
struct ip_ct_tcp tcp;
|
|
struct ip_ct_icmp icmp;
|
|
};
|
|
|
|
union ip_conntrack_expect_proto {
|
|
/* insert expect proto private data here */
|
|
};
|
|
|
|
/* Add protocol helper include file here */
|
|
#include <linux/netfilter_ipv4/ip_conntrack_h323.h>
|
|
#include <linux/netfilter_ipv4/ip_conntrack_pptp.h>
|
|
#include <linux/netfilter_ipv4/ip_conntrack_amanda.h>
|
|
#include <linux/netfilter_ipv4/ip_conntrack_ftp.h>
|
|
#include <linux/netfilter_ipv4/ip_conntrack_irc.h>
|
|
|
|
/* per conntrack: application helper private data */
|
|
union ip_conntrack_help {
|
|
/* insert conntrack helper private data (master) here */
|
|
struct ip_ct_h323_master ct_h323_info;
|
|
struct ip_ct_pptp_master ct_pptp_info;
|
|
struct ip_ct_ftp_master ct_ftp_info;
|
|
struct ip_ct_irc_master ct_irc_info;
|
|
};
|
|
|
|
#ifdef CONFIG_IP_NF_NAT_NEEDED
|
|
#include <linux/netfilter_ipv4/ip_nat.h>
|
|
#include <linux/netfilter_ipv4/ip_nat_pptp.h>
|
|
|
|
/* per conntrack: nat application helper private data */
|
|
union ip_conntrack_nat_help {
|
|
/* insert nat helper private data here */
|
|
struct ip_nat_pptp nat_pptp_info;
|
|
};
|
|
#endif
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/skbuff.h>
|
|
|
|
#ifdef CONFIG_NETFILTER_DEBUG
|
|
#define IP_NF_ASSERT(x) \
|
|
do { \
|
|
if (!(x)) \
|
|
/* Wooah! I'm tripping my conntrack in a frenzy of \
|
|
netplay... */ \
|
|
printk("NF_IP_ASSERT: %s:%i(%s)\n", \
|
|
__FILE__, __LINE__, __FUNCTION__); \
|
|
} while(0)
|
|
#else
|
|
#define IP_NF_ASSERT(x)
|
|
#endif
|
|
|
|
struct ip_conntrack_helper;
|
|
|
|
struct ip_conntrack
|
|
{
|
|
/* Usage count in here is 1 for hash table/destruct timer, 1 per skb,
|
|
plus 1 for any connection(s) we are `master' for */
|
|
struct nf_conntrack ct_general;
|
|
|
|
/* Have we seen traffic both ways yet? (bitset) */
|
|
unsigned long status;
|
|
|
|
/* Timer function; drops refcnt when it goes off. */
|
|
struct timer_list timeout;
|
|
|
|
#ifdef CONFIG_IP_NF_CT_ACCT
|
|
/* Accounting Information (same cache line as other written members) */
|
|
struct ip_conntrack_counter counters[IP_CT_DIR_MAX];
|
|
#endif
|
|
/* If we were expected by an expectation, this will be it */
|
|
struct ip_conntrack *master;
|
|
|
|
/* Current number of expected connections */
|
|
unsigned int expecting;
|
|
|
|
/* Unique ID that identifies this conntrack*/
|
|
unsigned int id;
|
|
|
|
/* Helper, if any. */
|
|
struct ip_conntrack_helper *helper;
|
|
|
|
/* Storage reserved for other modules: */
|
|
union ip_conntrack_proto proto;
|
|
|
|
union ip_conntrack_help help;
|
|
|
|
#ifdef CONFIG_IP_NF_NAT_NEEDED
|
|
struct {
|
|
struct ip_nat_info info;
|
|
union ip_conntrack_nat_help help;
|
|
#if defined(CONFIG_IP_NF_TARGET_MASQUERADE) || \
|
|
defined(CONFIG_IP_NF_TARGET_MASQUERADE_MODULE)
|
|
int masq_index;
|
|
#endif
|
|
} nat;
|
|
#endif /* CONFIG_IP_NF_NAT_NEEDED */
|
|
|
|
#if defined(CONFIG_IP_NF_CONNTRACK_MARK)
|
|
u_int32_t mark;
|
|
#endif
|
|
|
|
/* Traversed often, so hopefully in different cacheline to top */
|
|
/* These are my tuples; original and reply */
|
|
struct ip_conntrack_tuple_hash tuplehash[IP_CT_DIR_MAX];
|
|
};
|
|
|
|
struct ip_conntrack_expect
|
|
{
|
|
/* Internal linked list (global expectation list) */
|
|
struct list_head list;
|
|
|
|
/* We expect this tuple, with the following mask */
|
|
struct ip_conntrack_tuple tuple, mask;
|
|
|
|
/* Function to call after setup and insertion */
|
|
void (*expectfn)(struct ip_conntrack *new,
|
|
struct ip_conntrack_expect *this);
|
|
|
|
/* The conntrack of the master connection */
|
|
struct ip_conntrack *master;
|
|
|
|
/* Timer function; deletes the expectation. */
|
|
struct timer_list timeout;
|
|
|
|
/* Usage count. */
|
|
atomic_t use;
|
|
|
|
/* Unique ID */
|
|
unsigned int id;
|
|
|
|
/* Flags */
|
|
unsigned int flags;
|
|
|
|
#ifdef CONFIG_IP_NF_NAT_NEEDED
|
|
/* This is the original per-proto part, used to map the
|
|
* expected connection the way the recipient expects. */
|
|
union ip_conntrack_manip_proto saved_proto;
|
|
/* Direction relative to the master connection. */
|
|
enum ip_conntrack_dir dir;
|
|
#endif
|
|
};
|
|
|
|
#define IP_CT_EXPECT_PERMANENT 0x1
|
|
|
|
static inline struct ip_conntrack *
|
|
tuplehash_to_ctrack(const struct ip_conntrack_tuple_hash *hash)
|
|
{
|
|
return container_of(hash, struct ip_conntrack,
|
|
tuplehash[hash->tuple.dst.dir]);
|
|
}
|
|
|
|
/* get master conntrack via master expectation */
|
|
#define master_ct(conntr) (conntr->master)
|
|
|
|
/* Alter reply tuple (maybe alter helper). */
|
|
extern void
|
|
ip_conntrack_alter_reply(struct ip_conntrack *conntrack,
|
|
const struct ip_conntrack_tuple *newreply);
|
|
|
|
/* Is this tuple taken? (ignoring any belonging to the given
|
|
conntrack). */
|
|
extern int
|
|
ip_conntrack_tuple_taken(const struct ip_conntrack_tuple *tuple,
|
|
const struct ip_conntrack *ignored_conntrack);
|
|
|
|
/* Return conntrack_info and tuple hash for given skb. */
|
|
static inline struct ip_conntrack *
|
|
ip_conntrack_get(const struct sk_buff *skb, enum ip_conntrack_info *ctinfo)
|
|
{
|
|
*ctinfo = skb->nfctinfo;
|
|
return (struct ip_conntrack *)skb->nfct;
|
|
}
|
|
|
|
/* decrement reference count on a conntrack */
|
|
static inline void
|
|
ip_conntrack_put(struct ip_conntrack *ct)
|
|
{
|
|
IP_NF_ASSERT(ct);
|
|
nf_conntrack_put(&ct->ct_general);
|
|
}
|
|
|
|
extern int invert_tuplepr(struct ip_conntrack_tuple *inverse,
|
|
const struct ip_conntrack_tuple *orig);
|
|
|
|
extern void __ip_ct_refresh_acct(struct ip_conntrack *ct,
|
|
enum ip_conntrack_info ctinfo,
|
|
const struct sk_buff *skb,
|
|
unsigned long extra_jiffies,
|
|
int do_acct);
|
|
|
|
/* Refresh conntrack for this many jiffies and do accounting */
|
|
static inline void ip_ct_refresh_acct(struct ip_conntrack *ct,
|
|
enum ip_conntrack_info ctinfo,
|
|
const struct sk_buff *skb,
|
|
unsigned long extra_jiffies)
|
|
{
|
|
__ip_ct_refresh_acct(ct, ctinfo, skb, extra_jiffies, 1);
|
|
}
|
|
|
|
/* Refresh conntrack for this many jiffies */
|
|
static inline void ip_ct_refresh(struct ip_conntrack *ct,
|
|
const struct sk_buff *skb,
|
|
unsigned long extra_jiffies)
|
|
{
|
|
__ip_ct_refresh_acct(ct, 0, skb, extra_jiffies, 0);
|
|
}
|
|
|
|
/* These are for NAT. Icky. */
|
|
/* Update TCP window tracking data when NAT mangles the packet */
|
|
extern void ip_conntrack_tcp_update(struct sk_buff *skb,
|
|
struct ip_conntrack *conntrack,
|
|
enum ip_conntrack_dir dir);
|
|
|
|
/* Call me when a conntrack is destroyed. */
|
|
extern void (*ip_conntrack_destroyed)(struct ip_conntrack *conntrack);
|
|
|
|
/* Fake conntrack entry for untracked connections */
|
|
extern struct ip_conntrack ip_conntrack_untracked;
|
|
|
|
/* Returns new sk_buff, or NULL */
|
|
struct sk_buff *
|
|
ip_ct_gather_frags(struct sk_buff *skb, u_int32_t user);
|
|
|
|
/* Iterate over all conntracks: if iter returns true, it's deleted. */
|
|
extern void
|
|
ip_ct_iterate_cleanup(int (*iter)(struct ip_conntrack *i, void *data),
|
|
void *data);
|
|
|
|
extern struct ip_conntrack_helper *
|
|
__ip_conntrack_helper_find_byname(const char *);
|
|
extern struct ip_conntrack_helper *
|
|
ip_conntrack_helper_find_get(const struct ip_conntrack_tuple *tuple);
|
|
extern void ip_conntrack_helper_put(struct ip_conntrack_helper *helper);
|
|
|
|
extern struct ip_conntrack_protocol *
|
|
__ip_conntrack_proto_find(u_int8_t protocol);
|
|
extern struct ip_conntrack_protocol *
|
|
ip_conntrack_proto_find_get(u_int8_t protocol);
|
|
extern void ip_conntrack_proto_put(struct ip_conntrack_protocol *proto);
|
|
|
|
extern void ip_ct_remove_expectations(struct ip_conntrack *ct);
|
|
|
|
extern struct ip_conntrack *ip_conntrack_alloc(struct ip_conntrack_tuple *,
|
|
struct ip_conntrack_tuple *);
|
|
|
|
extern void ip_conntrack_free(struct ip_conntrack *ct);
|
|
|
|
extern void ip_conntrack_hash_insert(struct ip_conntrack *ct);
|
|
|
|
extern struct ip_conntrack_expect *
|
|
__ip_conntrack_expect_find(const struct ip_conntrack_tuple *tuple);
|
|
|
|
extern struct ip_conntrack_expect *
|
|
ip_conntrack_expect_find(const struct ip_conntrack_tuple *tuple);
|
|
|
|
extern struct ip_conntrack_tuple_hash *
|
|
__ip_conntrack_find(const struct ip_conntrack_tuple *tuple,
|
|
const struct ip_conntrack *ignored_conntrack);
|
|
|
|
extern void ip_conntrack_flush(void);
|
|
|
|
/* It's confirmed if it is, or has been in the hash table. */
|
|
static inline int is_confirmed(struct ip_conntrack *ct)
|
|
{
|
|
return test_bit(IPS_CONFIRMED_BIT, &ct->status);
|
|
}
|
|
|
|
static inline int is_dying(struct ip_conntrack *ct)
|
|
{
|
|
return test_bit(IPS_DYING_BIT, &ct->status);
|
|
}
|
|
|
|
extern unsigned int ip_conntrack_htable_size;
|
|
|
|
#define CONNTRACK_STAT_INC(count) (__get_cpu_var(ip_conntrack_stat).count++)
|
|
|
|
#ifdef CONFIG_IP_NF_CONNTRACK_EVENTS
|
|
#include <linux/notifier.h>
|
|
#include <linux/interrupt.h>
|
|
|
|
struct ip_conntrack_ecache {
|
|
struct ip_conntrack *ct;
|
|
unsigned int events;
|
|
};
|
|
DECLARE_PER_CPU(struct ip_conntrack_ecache, ip_conntrack_ecache);
|
|
|
|
#define CONNTRACK_ECACHE(x) (__get_cpu_var(ip_conntrack_ecache).x)
|
|
|
|
extern struct atomic_notifier_head ip_conntrack_chain;
|
|
extern struct atomic_notifier_head ip_conntrack_expect_chain;
|
|
|
|
static inline int ip_conntrack_register_notifier(struct notifier_block *nb)
|
|
{
|
|
return atomic_notifier_chain_register(&ip_conntrack_chain, nb);
|
|
}
|
|
|
|
static inline int ip_conntrack_unregister_notifier(struct notifier_block *nb)
|
|
{
|
|
return atomic_notifier_chain_unregister(&ip_conntrack_chain, nb);
|
|
}
|
|
|
|
static inline int
|
|
ip_conntrack_expect_register_notifier(struct notifier_block *nb)
|
|
{
|
|
return atomic_notifier_chain_register(&ip_conntrack_expect_chain, nb);
|
|
}
|
|
|
|
static inline int
|
|
ip_conntrack_expect_unregister_notifier(struct notifier_block *nb)
|
|
{
|
|
return atomic_notifier_chain_unregister(&ip_conntrack_expect_chain,
|
|
nb);
|
|
}
|
|
|
|
extern void ip_ct_deliver_cached_events(const struct ip_conntrack *ct);
|
|
extern void __ip_ct_event_cache_init(struct ip_conntrack *ct);
|
|
|
|
static inline void
|
|
ip_conntrack_event_cache(enum ip_conntrack_events event,
|
|
const struct sk_buff *skb)
|
|
{
|
|
struct ip_conntrack *ct = (struct ip_conntrack *)skb->nfct;
|
|
struct ip_conntrack_ecache *ecache;
|
|
|
|
local_bh_disable();
|
|
ecache = &__get_cpu_var(ip_conntrack_ecache);
|
|
if (ct != ecache->ct)
|
|
__ip_ct_event_cache_init(ct);
|
|
ecache->events |= event;
|
|
local_bh_enable();
|
|
}
|
|
|
|
static inline void ip_conntrack_event(enum ip_conntrack_events event,
|
|
struct ip_conntrack *ct)
|
|
{
|
|
if (is_confirmed(ct) && !is_dying(ct))
|
|
atomic_notifier_call_chain(&ip_conntrack_chain, event, ct);
|
|
}
|
|
|
|
static inline void
|
|
ip_conntrack_expect_event(enum ip_conntrack_expect_events event,
|
|
struct ip_conntrack_expect *exp)
|
|
{
|
|
atomic_notifier_call_chain(&ip_conntrack_expect_chain, event, exp);
|
|
}
|
|
#else /* CONFIG_IP_NF_CONNTRACK_EVENTS */
|
|
static inline void ip_conntrack_event_cache(enum ip_conntrack_events event,
|
|
const struct sk_buff *skb) {}
|
|
static inline void ip_conntrack_event(enum ip_conntrack_events event,
|
|
struct ip_conntrack *ct) {}
|
|
static inline void ip_ct_deliver_cached_events(const struct ip_conntrack *ct) {}
|
|
static inline void
|
|
ip_conntrack_expect_event(enum ip_conntrack_expect_events event,
|
|
struct ip_conntrack_expect *exp) {}
|
|
#endif /* CONFIG_IP_NF_CONNTRACK_EVENTS */
|
|
|
|
#ifdef CONFIG_IP_NF_NAT_NEEDED
|
|
static inline int ip_nat_initialized(struct ip_conntrack *conntrack,
|
|
enum ip_nat_manip_type manip)
|
|
{
|
|
if (manip == IP_NAT_MANIP_SRC)
|
|
return test_bit(IPS_SRC_NAT_DONE_BIT, &conntrack->status);
|
|
return test_bit(IPS_DST_NAT_DONE_BIT, &conntrack->status);
|
|
}
|
|
#endif /* CONFIG_IP_NF_NAT_NEEDED */
|
|
|
|
#endif /* __KERNEL__ */
|
|
#endif /* _IP_CONNTRACK_H */
|