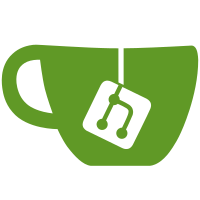
Thru series of refactorings functions were being renamed but not moved to map.c to reduce patch noise, now lets have them in the same place so that use of the symbol system by tools can be constrained to building and linking fewer source files: symbol.c, map.c and rbtree.c. Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com> Cc: Frédéric Weisbecker <fweisbec@gmail.com> Cc: Mike Galbraith <efault@gmx.de> Cc: Peter Zijlstra <a.p.zijlstra@chello.nl> Cc: Paul Mackerras <paulus@samba.org> LKML-Reference: <1269557941-15617-3-git-send-email-acme@infradead.org> Signed-off-by: Ingo Molnar <mingo@elte.hu>
317 lines
6.5 KiB
C
317 lines
6.5 KiB
C
#include "symbol.h"
|
|
#include <limits.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
#include <stdio.h>
|
|
#include "map.h"
|
|
|
|
const char *map_type__name[MAP__NR_TYPES] = {
|
|
[MAP__FUNCTION] = "Functions",
|
|
[MAP__VARIABLE] = "Variables",
|
|
};
|
|
|
|
static inline int is_anon_memory(const char *filename)
|
|
{
|
|
return strcmp(filename, "//anon") == 0;
|
|
}
|
|
|
|
static int strcommon(const char *pathname, char *cwd, int cwdlen)
|
|
{
|
|
int n = 0;
|
|
|
|
while (n < cwdlen && pathname[n] == cwd[n])
|
|
++n;
|
|
|
|
return n;
|
|
}
|
|
|
|
void map__init(struct map *self, enum map_type type,
|
|
u64 start, u64 end, u64 pgoff, struct dso *dso)
|
|
{
|
|
self->type = type;
|
|
self->start = start;
|
|
self->end = end;
|
|
self->pgoff = pgoff;
|
|
self->dso = dso;
|
|
self->map_ip = map__map_ip;
|
|
self->unmap_ip = map__unmap_ip;
|
|
RB_CLEAR_NODE(&self->rb_node);
|
|
}
|
|
|
|
struct map *map__new(u64 start, u64 len, u64 pgoff, u32 pid, char *filename,
|
|
enum map_type type, char *cwd, int cwdlen)
|
|
{
|
|
struct map *self = malloc(sizeof(*self));
|
|
|
|
if (self != NULL) {
|
|
char newfilename[PATH_MAX];
|
|
struct dso *dso;
|
|
int anon;
|
|
|
|
if (cwd) {
|
|
int n = strcommon(filename, cwd, cwdlen);
|
|
|
|
if (n == cwdlen) {
|
|
snprintf(newfilename, sizeof(newfilename),
|
|
".%s", filename + n);
|
|
filename = newfilename;
|
|
}
|
|
}
|
|
|
|
anon = is_anon_memory(filename);
|
|
|
|
if (anon) {
|
|
snprintf(newfilename, sizeof(newfilename), "/tmp/perf-%d.map", pid);
|
|
filename = newfilename;
|
|
}
|
|
|
|
dso = dsos__findnew(filename);
|
|
if (dso == NULL)
|
|
goto out_delete;
|
|
|
|
map__init(self, type, start, start + len, pgoff, dso);
|
|
|
|
if (anon) {
|
|
set_identity:
|
|
self->map_ip = self->unmap_ip = identity__map_ip;
|
|
} else if (strcmp(filename, "[vdso]") == 0) {
|
|
dso__set_loaded(dso, self->type);
|
|
goto set_identity;
|
|
}
|
|
}
|
|
return self;
|
|
out_delete:
|
|
free(self);
|
|
return NULL;
|
|
}
|
|
|
|
void map__delete(struct map *self)
|
|
{
|
|
free(self);
|
|
}
|
|
|
|
void map__fixup_start(struct map *self)
|
|
{
|
|
struct rb_root *symbols = &self->dso->symbols[self->type];
|
|
struct rb_node *nd = rb_first(symbols);
|
|
if (nd != NULL) {
|
|
struct symbol *sym = rb_entry(nd, struct symbol, rb_node);
|
|
self->start = sym->start;
|
|
}
|
|
}
|
|
|
|
void map__fixup_end(struct map *self)
|
|
{
|
|
struct rb_root *symbols = &self->dso->symbols[self->type];
|
|
struct rb_node *nd = rb_last(symbols);
|
|
if (nd != NULL) {
|
|
struct symbol *sym = rb_entry(nd, struct symbol, rb_node);
|
|
self->end = sym->end;
|
|
}
|
|
}
|
|
|
|
#define DSO__DELETED "(deleted)"
|
|
|
|
int map__load(struct map *self, symbol_filter_t filter)
|
|
{
|
|
const char *name = self->dso->long_name;
|
|
int nr;
|
|
|
|
if (dso__loaded(self->dso, self->type))
|
|
return 0;
|
|
|
|
nr = dso__load(self->dso, self, filter);
|
|
if (nr < 0) {
|
|
if (self->dso->has_build_id) {
|
|
char sbuild_id[BUILD_ID_SIZE * 2 + 1];
|
|
|
|
build_id__sprintf(self->dso->build_id,
|
|
sizeof(self->dso->build_id),
|
|
sbuild_id);
|
|
pr_warning("%s with build id %s not found",
|
|
name, sbuild_id);
|
|
} else
|
|
pr_warning("Failed to open %s", name);
|
|
|
|
pr_warning(", continuing without symbols\n");
|
|
return -1;
|
|
} else if (nr == 0) {
|
|
const size_t len = strlen(name);
|
|
const size_t real_len = len - sizeof(DSO__DELETED);
|
|
|
|
if (len > sizeof(DSO__DELETED) &&
|
|
strcmp(name + real_len + 1, DSO__DELETED) == 0) {
|
|
pr_warning("%.*s was updated, restart the long "
|
|
"running apps that use it!\n",
|
|
(int)real_len, name);
|
|
} else {
|
|
pr_warning("no symbols found in %s, maybe install "
|
|
"a debug package?\n", name);
|
|
}
|
|
|
|
return -1;
|
|
}
|
|
/*
|
|
* Only applies to the kernel, as its symtabs aren't relative like the
|
|
* module ones.
|
|
*/
|
|
if (self->dso->kernel)
|
|
map__reloc_vmlinux(self);
|
|
|
|
return 0;
|
|
}
|
|
|
|
struct symbol *map__find_symbol(struct map *self, u64 addr,
|
|
symbol_filter_t filter)
|
|
{
|
|
if (map__load(self, filter) < 0)
|
|
return NULL;
|
|
|
|
return dso__find_symbol(self->dso, self->type, addr);
|
|
}
|
|
|
|
struct symbol *map__find_symbol_by_name(struct map *self, const char *name,
|
|
symbol_filter_t filter)
|
|
{
|
|
if (map__load(self, filter) < 0)
|
|
return NULL;
|
|
|
|
if (!dso__sorted_by_name(self->dso, self->type))
|
|
dso__sort_by_name(self->dso, self->type);
|
|
|
|
return dso__find_symbol_by_name(self->dso, self->type, name);
|
|
}
|
|
|
|
struct map *map__clone(struct map *self)
|
|
{
|
|
struct map *map = malloc(sizeof(*self));
|
|
|
|
if (!map)
|
|
return NULL;
|
|
|
|
memcpy(map, self, sizeof(*self));
|
|
|
|
return map;
|
|
}
|
|
|
|
int map__overlap(struct map *l, struct map *r)
|
|
{
|
|
if (l->start > r->start) {
|
|
struct map *t = l;
|
|
l = r;
|
|
r = t;
|
|
}
|
|
|
|
if (l->end > r->start)
|
|
return 1;
|
|
|
|
return 0;
|
|
}
|
|
|
|
size_t map__fprintf(struct map *self, FILE *fp)
|
|
{
|
|
return fprintf(fp, " %Lx-%Lx %Lx %s\n",
|
|
self->start, self->end, self->pgoff, self->dso->name);
|
|
}
|
|
|
|
/*
|
|
* objdump wants/reports absolute IPs for ET_EXEC, and RIPs for ET_DYN.
|
|
* map->dso->adjust_symbols==1 for ET_EXEC-like cases.
|
|
*/
|
|
u64 map__rip_2objdump(struct map *map, u64 rip)
|
|
{
|
|
u64 addr = map->dso->adjust_symbols ?
|
|
map->unmap_ip(map, rip) : /* RIP -> IP */
|
|
rip;
|
|
return addr;
|
|
}
|
|
|
|
u64 map__objdump_2ip(struct map *map, u64 addr)
|
|
{
|
|
u64 ip = map->dso->adjust_symbols ?
|
|
addr :
|
|
map->unmap_ip(map, addr); /* RIP -> IP */
|
|
return ip;
|
|
}
|
|
|
|
struct symbol *map_groups__find_symbol(struct map_groups *self,
|
|
enum map_type type, u64 addr,
|
|
symbol_filter_t filter)
|
|
{
|
|
struct map *map = map_groups__find(self, type, addr);
|
|
|
|
if (map != NULL)
|
|
return map__find_symbol(map, map->map_ip(map, addr), filter);
|
|
|
|
return NULL;
|
|
}
|
|
|
|
static u64 map__reloc_map_ip(struct map *map, u64 ip)
|
|
{
|
|
return ip + (s64)map->pgoff;
|
|
}
|
|
|
|
static u64 map__reloc_unmap_ip(struct map *map, u64 ip)
|
|
{
|
|
return ip - (s64)map->pgoff;
|
|
}
|
|
|
|
void map__reloc_vmlinux(struct map *self)
|
|
{
|
|
struct kmap *kmap = map__kmap(self);
|
|
s64 reloc;
|
|
|
|
if (!kmap->ref_reloc_sym || !kmap->ref_reloc_sym->unrelocated_addr)
|
|
return;
|
|
|
|
reloc = (kmap->ref_reloc_sym->unrelocated_addr -
|
|
kmap->ref_reloc_sym->addr);
|
|
|
|
if (!reloc)
|
|
return;
|
|
|
|
self->map_ip = map__reloc_map_ip;
|
|
self->unmap_ip = map__reloc_unmap_ip;
|
|
self->pgoff = reloc;
|
|
}
|
|
|
|
void maps__insert(struct rb_root *maps, struct map *map)
|
|
{
|
|
struct rb_node **p = &maps->rb_node;
|
|
struct rb_node *parent = NULL;
|
|
const u64 ip = map->start;
|
|
struct map *m;
|
|
|
|
while (*p != NULL) {
|
|
parent = *p;
|
|
m = rb_entry(parent, struct map, rb_node);
|
|
if (ip < m->start)
|
|
p = &(*p)->rb_left;
|
|
else
|
|
p = &(*p)->rb_right;
|
|
}
|
|
|
|
rb_link_node(&map->rb_node, parent, p);
|
|
rb_insert_color(&map->rb_node, maps);
|
|
}
|
|
|
|
struct map *maps__find(struct rb_root *maps, u64 ip)
|
|
{
|
|
struct rb_node **p = &maps->rb_node;
|
|
struct rb_node *parent = NULL;
|
|
struct map *m;
|
|
|
|
while (*p != NULL) {
|
|
parent = *p;
|
|
m = rb_entry(parent, struct map, rb_node);
|
|
if (ip < m->start)
|
|
p = &(*p)->rb_left;
|
|
else if (ip > m->end)
|
|
p = &(*p)->rb_right;
|
|
else
|
|
return m;
|
|
}
|
|
|
|
return NULL;
|
|
}
|