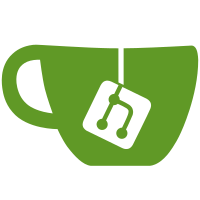
Cleanup of page table allocators, using generic folded PMD and PUD helpers. TLB flushing operations are moved to a more sensible spot. The page fault handler is also optimized slightly, we no longer waste cycles on IRQ disabling for flushing of the page from the ITLB, since we're already under CLI protection by the initial exception handler. Signed-off-by: Paul Mundt <lethal@linux-sh.org>
60 lines
1.4 KiB
C
60 lines
1.4 KiB
C
#ifndef __ASM_SH_PGALLOC_H
|
|
#define __ASM_SH_PGALLOC_H
|
|
|
|
#define pmd_populate_kernel(mm, pmd, pte) \
|
|
set_pmd(pmd, __pmd(_PAGE_TABLE + __pa(pte)))
|
|
|
|
static inline void pmd_populate(struct mm_struct *mm, pmd_t *pmd,
|
|
struct page *pte)
|
|
{
|
|
set_pmd(pmd, __pmd(_PAGE_TABLE + page_to_phys(pte)));
|
|
}
|
|
|
|
/*
|
|
* Allocate and free page tables.
|
|
*/
|
|
static inline pgd_t *pgd_alloc(struct mm_struct *mm)
|
|
{
|
|
return (pgd_t *)__get_free_page(GFP_KERNEL | __GFP_REPEAT | __GFP_ZERO);
|
|
}
|
|
|
|
static inline void pgd_free(pgd_t *pgd)
|
|
{
|
|
free_page((unsigned long)pgd);
|
|
}
|
|
|
|
static inline pte_t *pte_alloc_one_kernel(struct mm_struct *mm,
|
|
unsigned long address)
|
|
{
|
|
return (pte_t *)__get_free_page(GFP_KERNEL | __GFP_REPEAT | __GFP_ZERO);
|
|
}
|
|
|
|
static inline struct page *pte_alloc_one(struct mm_struct *mm,
|
|
unsigned long address)
|
|
{
|
|
return alloc_page(GFP_KERNEL | __GFP_REPEAT | __GFP_ZERO);
|
|
}
|
|
|
|
static inline void pte_free_kernel(pte_t *pte)
|
|
{
|
|
free_page((unsigned long)pte);
|
|
}
|
|
|
|
static inline void pte_free(struct page *pte)
|
|
{
|
|
__free_page(pte);
|
|
}
|
|
|
|
#define __pte_free_tlb(tlb,pte) tlb_remove_page((tlb),(pte))
|
|
|
|
/*
|
|
* allocating and freeing a pmd is trivial: the 1-entry pmd is
|
|
* inside the pgd, so has no extra memory associated with it.
|
|
*/
|
|
|
|
#define pmd_free(x) do { } while (0)
|
|
#define __pmd_free_tlb(tlb,x) do { } while (0)
|
|
#define check_pgt_cache() do { } while (0)
|
|
|
|
#endif /* __ASM_SH_PGALLOC_H */
|